자바스크립트 프로토타입(Prototype)이란? 객체 지향 프로그래밍
자바스크립트는 객체 지향 프로그래밍을 지원하는 언어로, 프로토타입(prototype)이라는 독특한 개념을 가지고 있습니다. 프로토타입은 객체 지향 프로그래밍의 기초 개념을 이해하는 데 중요한 역할을 합니다. 이 글에서는 자바스크립트 프로토타입의 기본 개념부터 고급 활용법까지 체계적으로 알아보겠습니다.
1. 프로토타입이란?
프로토타입은 자바스크립트에서 객체가 다른 객체로부터 속성과 메서드를 상속받을 수 있게 하는 메커니즘입니다. 모든 자바스크립트 객체는 숨겨진 [[Prototype]]
속성을 가지고 있으며, 이는 다른 객체를 가리킵니다. 이로 인해 객체는 부모 객체의 속성과 메서드를 사용할 수 있습니다.
2. 프로토타입 체인
프로토타입 체인은 객체의 프로토타입이 또 다른 객체의 프로토타입을 참조하는 구조입니다. 이 체인을 통해 객체는 상속된 속성과 메서드를 찾을 수 있습니다. 프로토타입 체인의 끝은 항상 null
입니다.
function Person(name) {
this.name = name;
}
Person.prototype.sayHello = function() {
console.log('Hello, ' + this.name);
};
const person1 = new Person('Alice');
person1.sayHello(); // Hello, Alice
3. 프로토타입 상속
자바스크립트에서는 객체가 다른 객체의 속성과 메서드를 상속받을 수 있습니다. 이를 통해 코드의 재사용성을 높이고, 효율적인 객체 지향 프로그래밍을 구현할 수 있습니다.
function Animal(name) {
this.name = name;
}
Animal.prototype.speak = function() {
console.log(this.name + ' makes a sound.');
};
function Dog(name, breed) {
Animal.call(this, name);
this.breed = breed;
}
Dog.prototype = Object.create(Animal.prototype);
Dog.prototype.constructor = Dog;
Dog.prototype.speak = function() {
console.log(this.name + ' barks.');
};
const dog1 = new Dog('Rex', 'German Shepherd');
dog1.speak(); // Rex barks.
4. 프로토타입 메서드
프로토타입 메서드는 객체 인스턴스가 생성될 때마다 해당 메서드를 복사하지 않고, 프로토타입 체인을 통해 공유됩니다. 이를 통해 메모리 사용을 줄이고, 성능을 향상시킬 수 있습니다.
function Car(model) {
this.model = model;
}
Car.prototype.drive = function() {
console.log(this.model + ' is driving.');
};
const car1 = new Car('Toyota');
const car2 = new Car('Honda');
car1.drive(); // Toyota is driving.
car2.drive(); // Honda is driving.
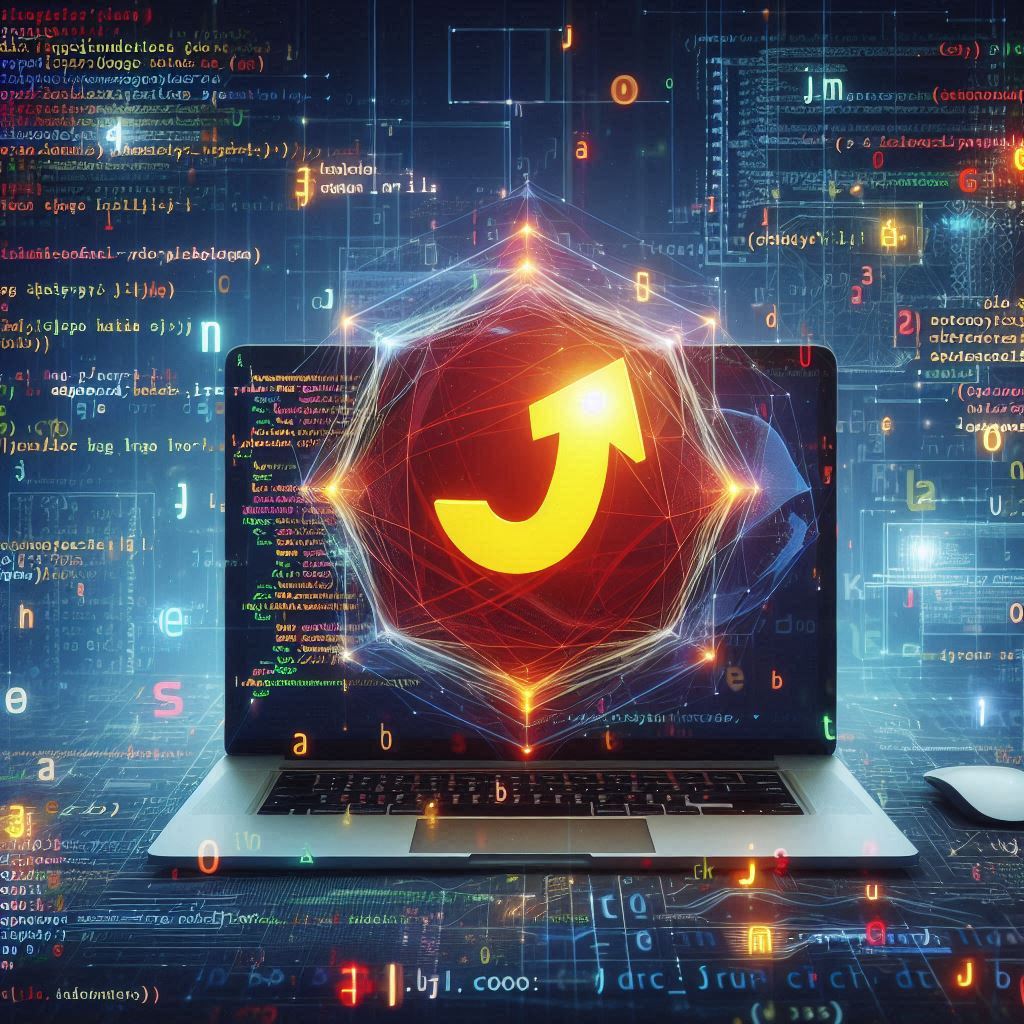
5. 프로토타입 활용 예제
프로토타입을 활용한 다양한 예제를 통해 실전에서 어떻게 사용되는지 알아보겠습니다.
function Book(title, author) {
this.title = title;
this.author = author;
}
Book.prototype.getDetails = function() {
return this.title + ' by ' + this.author;
};
const book1 = new Book('1984', 'George Orwell');
const book2 = new Book('Brave New World', 'Aldous Huxley');
console.log(book1.getDetails()); // 1984 by George Orwell
console.log(book2.getDetails()); // Brave New World by Aldous Huxley
6. 결론
자바스크립트의 프로토타입은 객체 지향 프로그래밍의 핵심 개념 중 하나입니다. 프로토타입을 이해하고 활용함으로써 코드의 재사용성을 높이고, 효율적인 프로그램을 작성할 수 있습니다. 이 가이드를 통해 프로토타입의 기본 개념과 활용법을 익히고, 실전에서 효과적으로 활용해 보세요.
'프로그래밍 언어 > 자바스크립트' 카테고리의 다른 글
자바스크립트 클래스(class) 기초 개념과 실전 예제 (0) | 2024.07.30 |
---|---|
JavaScript 프로토타입(Prototype) 상속 체인 확장 이해하기 (0) | 2024.07.28 |
자바스크립트 객체와 배열의 메서드 활용법 (0) | 2024.07.26 |
자바스크립트(JavaScript) 배열 생성, 조작, 활용 방법 (0) | 2024.07.25 |
자바스크립트(JavaScript) 객체 생성, 접근, 수정, 삭제 방법 (0) | 2024.07.24 |