파이썬 문자열 메서드 기본 사용 방법과 실전 예제
파이썬에서 문자열은 가장 기본적이면서도 자주 사용하는 데이터 타입 중 하나입니다. 문자열을 다루는 다양한 메서드를 잘 활용하면, 복잡한 텍스트 처리 작업도 손쉽게 해결할 수 있습니다. 이 글에서는 파이썬 문자열 메서드의 기초 개념부터 고급 활용법까지 체계적으로 살펴보겠습니다.
1. 문자열 기본 메서드
파이썬에서는 문자열을 다루기 위한 다양한 기본 메서드를 제공합니다. 대표적인 예로 upper()
, lower()
, strip()
등이 있습니다.
text = " Hello, Python! "
print(text.upper()) # " HELLO, PYTHON! "
print(text.lower()) # " hello, python! "
print(text.strip()) # "Hello, Python!"
2. 문자열 탐색 및 치환
문자열 내 특정 문자를 찾거나 다른 문자로 치환할 때 사용하는 메서드로는 find()
, replace()
등이 있습니다.
text = "Hello, Python!"
print(text.find("Python")) # 7
print(text.replace("Python", "World")) # "Hello, World!"
3. 문자열 분할 및 결합
문자열을 분할하거나 결합하는 작업은 split()
과 join()
메서드를 사용합니다.
text = "apple,banana,cherry"
fruits = text.split(",")
print(fruits) # ['apple', 'banana', 'cherry']
joined_text = " ".join(fruits)
print(joined_text) # "apple banana cherry"
4. 문자열 형식화
문자열을 형식화하는 방법에는 format()
메서드와 f-문자열이 있습니다. 이를 통해 가독성 높은 문자열을 쉽게 만들 수 있습니다.
name = "Alice"
age = 25
print("My name is {} and I am {} years old.".format(name, age))
# "My name is Alice and I am 25 years old."
print(f"My name is {name} and I am {age} years old.")
# "My name is Alice and I am 25 years old."
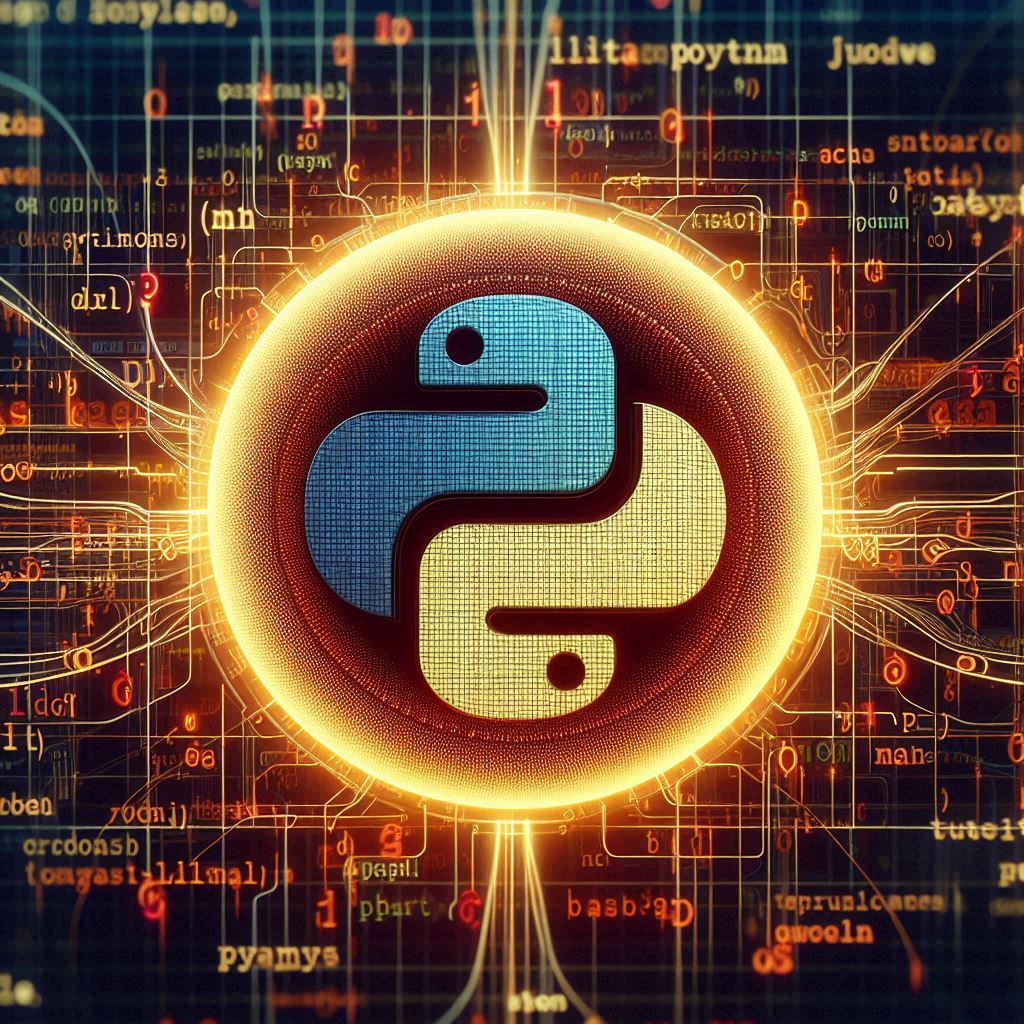
5. 고급 문자열 메서드
고급 문자열 메서드에는 startswith()
, endswith()
, isnumeric()
등이 있습니다. 이러한 메서드를 사용하면 문자열의 특정 조건을 쉽게 검사할 수 있습니다.
text = "12345"
print(text.startswith("123")) # True
print(text.endswith("45")) # True
print(text.isnumeric()) # True
6. 실전 예제
위에서 배운 문자열 메서드를 종합적으로 활용하여, 실전 예제를 통해 문자열 처리를 더욱 명확하게 이해해 보겠습니다.
text = " Welcome to the world of Python! "
# 공백 제거 및 소문자로 변환
processed_text = text.strip().lower()
# 특정 단어 찾기
if "python" in processed_text:
print("The word 'python' is in the text.")
# 단어 치환
processed_text = processed_text.replace("world", "universe")
print(processed_text) # "welcome to the universe of python!"
7. 결론
이번 글에서는 파이썬 문자열 메서드의 다양한 활용법과 예제를 통해 문자열 처리를 효과적으로 수행하는 방법을 알아보았습니다. 문자열 메서드를 잘 활용하면 텍스트 데이터 처리 작업을 효율적으로 수행할 수 있습니다. 실전 예제를 통해 배운 내용을 직접 적용해 보세요.
'프로그래밍 언어 > 파이썬' 카테고리의 다른 글
파이썬(Python) 파일 읽기, 다양한 방법과 실전 예제 (0) | 2024.07.26 |
---|---|
파이썬(Python) 정규 표현식 기초부터 고급 활용까지 (0) | 2024.07.25 |
파이썬(Python) 문자열 슬라이싱, 연결, 포맷팅 방법 (0) | 2024.07.23 |
파이썬(Python) 집합(set) 기본 개념, 고급 연산, 실전 예제 (0) | 2024.07.22 |
파이썬(Python) 딕셔너리 기초 개념과 실전 예제 (0) | 2024.07.21 |